Suppose you are given a task to generate average of 50 numbers. So, each time you run the program you have to type 50 numbers from your keyboard and then your program will do the rest. But doesn’t it sound like a tiresome task to type 50 numbers? Wouldn’t it be better if the numbers were saved in a text file so that your program can have those numbers directly from reading that file and giving you the average? Obviously yes, that would save you a lot of time.
However, no worries! In C, we can easily do this. C is so efficient in reading input from a file or writing output to a file. This is known as file handling. Let’s see how we can create a file at first.
File creation
Befor going straight into coding, let me give you some keypoints.
Keypoint 1: At first you need to have a location of your file (existing/ newly created). Without the location, it will do no work.
Keypoint 2: You need to mention the mode of your file. For example: if you want to write a file, the mode will be “w”. And I guess you guess it perfect the mode for reading, which is “r”.
Keypoint 3: You need to have a file pointer. This pointer is a must and through this pointer you will read from and write into a file.
So we have our required basics. Now let’s see how we can implement the basic into code.
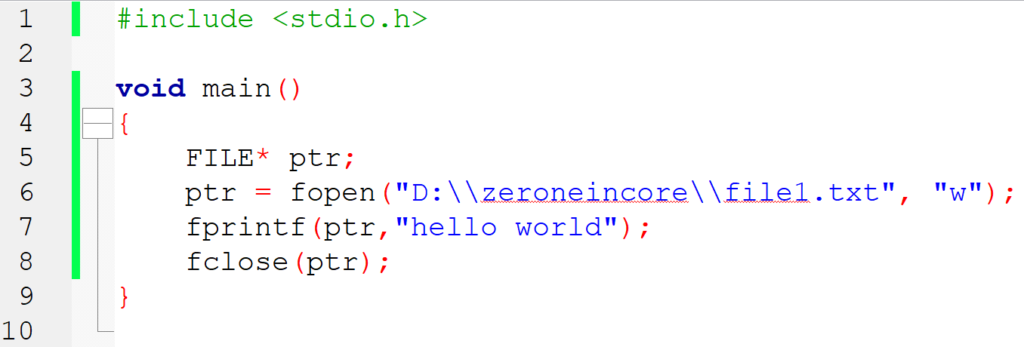
Let’s analyze the code
Line 5-6: Line 6 creates a file named file1.txt at my desired location D:\zeroneincore in write mode (as I want to write something into this file) and the file pointer ptr (created at line 5) is pointing at the starting location of the file through fopen() built-in function. So, anything you write will be at the starting location of the file1.txt file.
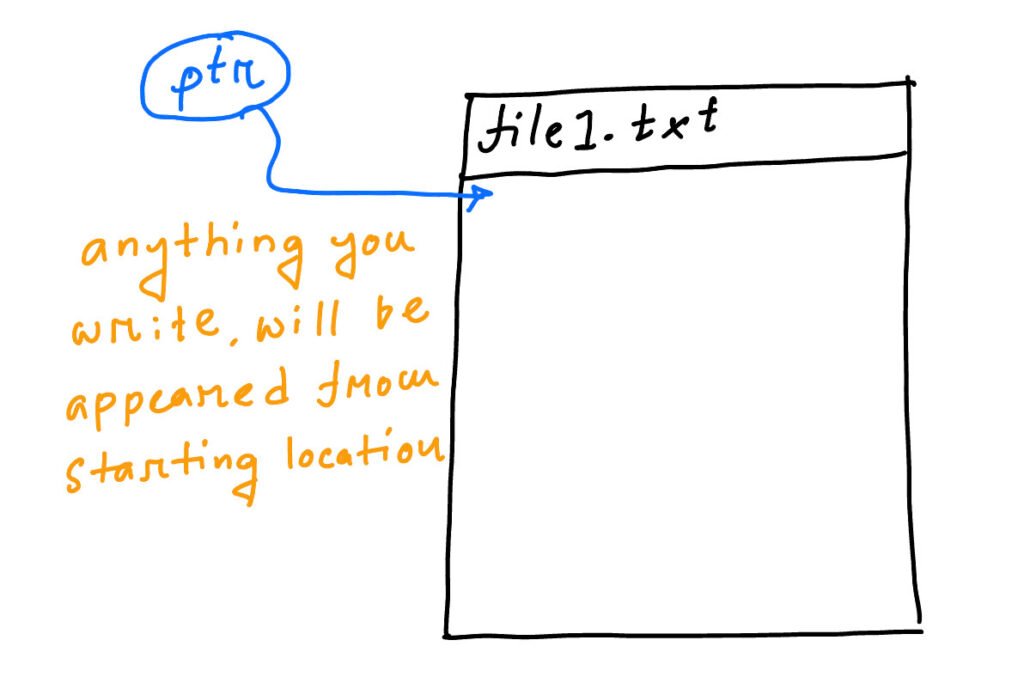
NB: If the file1.txt does not exist at location D:\zeroneincore, no worries. Your program will create one.
NB: If the file1.txt exists at location D:\zeroneincore, all the old data written in it will be erased by your given data. So be careful if you don’t wish to lose anything
Line 7: The fprintf() built-in function is used to write into the file. It takes 2 parameters
- the file pointer through which it writes
- and the text you wish to put inside the file
Line 8: The fclose() is used to close a file. It takes a file pointer as a parameter. Its task is to let the file pointer take leave from its job (for example, pointing a file/ reading/ writing). Closing a file is a good practice as it cleans up unnecessary memory space.
I hope I have explained it clear enough. If you want to learn the advanced topics in C, you can check out the book C: The Complete Reference by Herbert Schildt.
Thank you!