After we are done with writing into a file, there is no surprise this blog is going to be on how we can read from a file. So let’s get started:
Before reading from a file, you need to keep in mind 5 things
- The file must exist on your pc (but in writing it was not necessary, remember?). If not, the reading operation will not be done.
- The location of the file to read. Suppose we want to read the file below. The location will be D:\\zeroneincore\\file.txt.
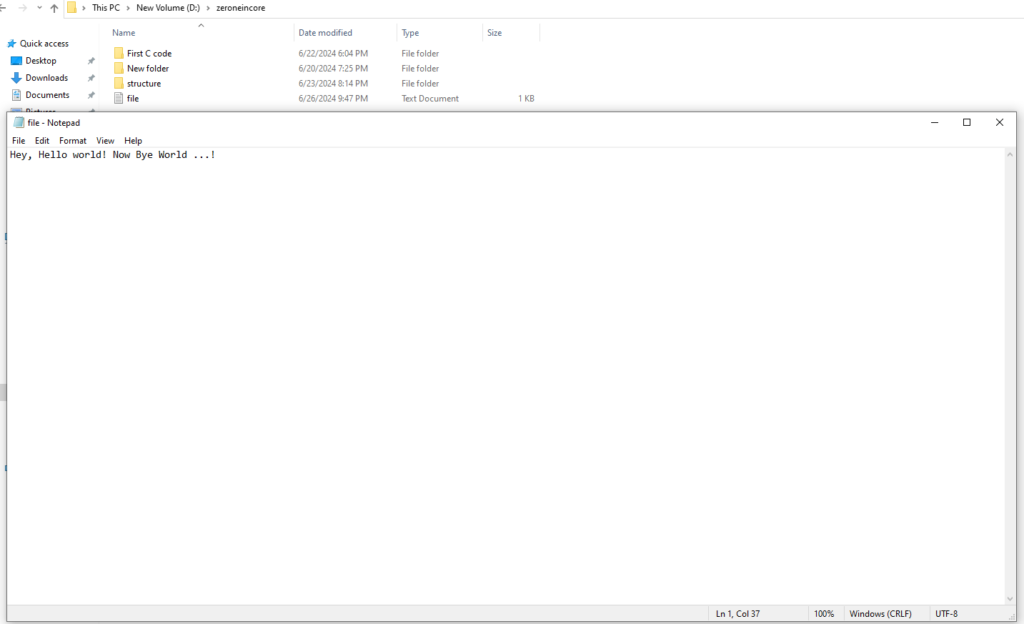
- Define the mode of your operation. For reading, it will simply be “r”.
- The file pointer through which the reading will be done.
- Finally, a string variable large enough to hold all the contents of your file. If the string is not large enough, we might encounter errors while reading or lose the contents of the file.
Now let’s see how we can make it into code.
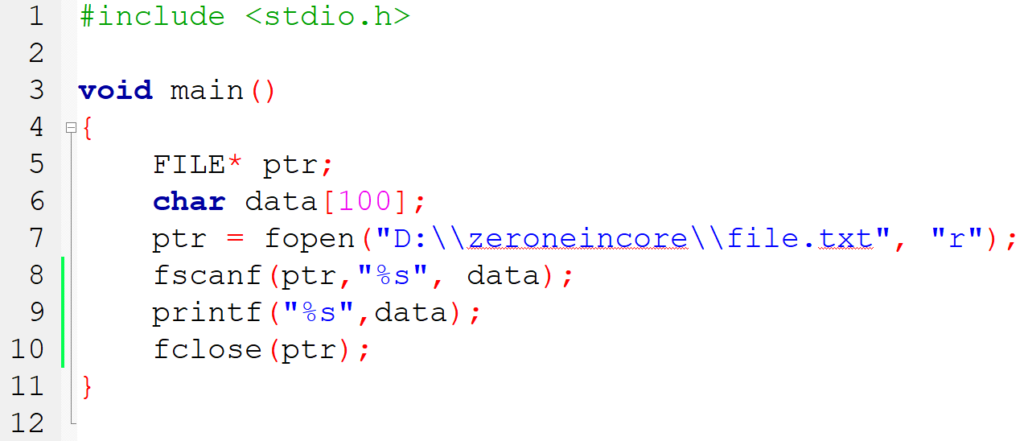
The code is almost similar to that of file writing, except
- There is a string data[100] to hold the contents coming from our file file.txt at line 6.
- The mode is “r” at line 7 for reading purpose.
- At line 8, fscanf() is a built-in function which stores the content of a file into a variable (here, in data) through the file pointer ptr.
- Finally, we print the values of data on the output screen at line 9.
If we run the code, we will see a similar output given below
Hey,
Only Hey, is shown on the output screen. Where are the rest of the contents? Are the contents truncated due to not enough large? No, because we have assigned large eniugh space for data (100 characters). So why this problem?
The answer is the fscanf() can read all the characters until it encounter a space. If it encounters a space, it stops reading. In simple words, it can read word by word. That’s why we got only Hey, .
To read all of the contents, we need to call fscanf() multiple times. How many times? The answer is until it reaches to End-of-file (EOF) i.e. when no characters are left to read in a file. Take a look at the updated code.
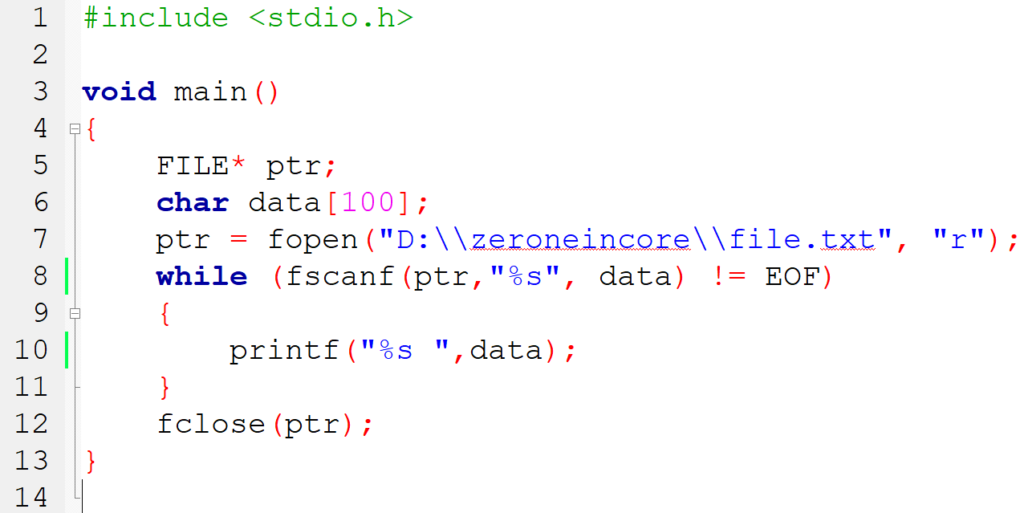
Just one more thing, don’t forget to put the space after %s at line 10, otherwise you will get a output like “Hey,Helloworld!NowByeWorld …!” as it only reads word by word.
I hope I have made you understand how you can read from a file. You can also take a look at C Programming Absolute Beginner’s Guide By Greg Perry and Dean Miller. It has some amazing explanation.
Thank you!