We have successfully declared and initialized a struct variable. However, the next thing is, how can we modify the struct members at a later point in our code? The answer is pretty straight-forward: by using the dot (.) operator you can access each and every member variables and modify their values. Take a look at the code below:
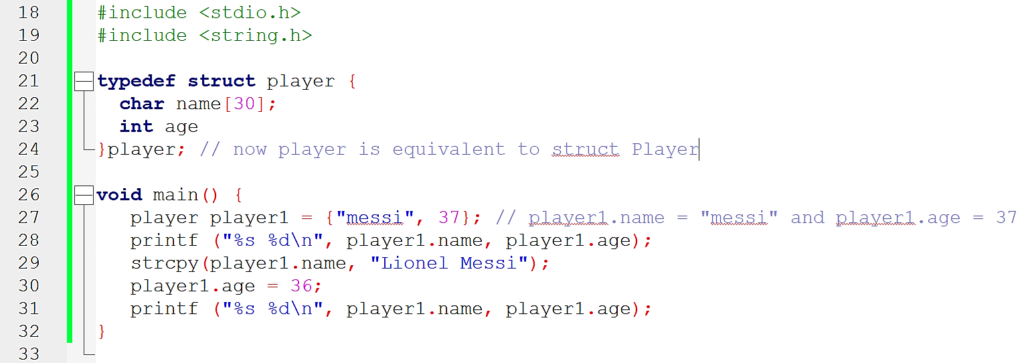
Look at two changes made in this code:
- Line 30: To change the age of player1 from 37 to 36, we’ve just accessed the age by player1.age and assigned it to a new value 36.
- Line 29: To change the name of player1 from “messi” to “Lionel Messi”, we took the help of a built-in function strcpy(), and assigned a new value “Lionel Messi” to player1.name.
However, one question should have appeared in your mind. Why did we use strcpy()? Couldn’t we just do it by player1.name = “Lionel Messi” ?
The answer is NO. Because, you can directly initialize a character array with a string literal at the time of declaration,
char c[30] = “asdasdsa”; // Valid;
but you cannot use the assignment operator to assign a string to an already-declared array
char c[30] = “asdasdsa”;
c = “new string” // Invalid
As in our example, the player1.name array is already declared and initialized with value “messi” at line 27, so you can not use the equal (=) operator at a later point.
I hope this explains good enough. Thank you.